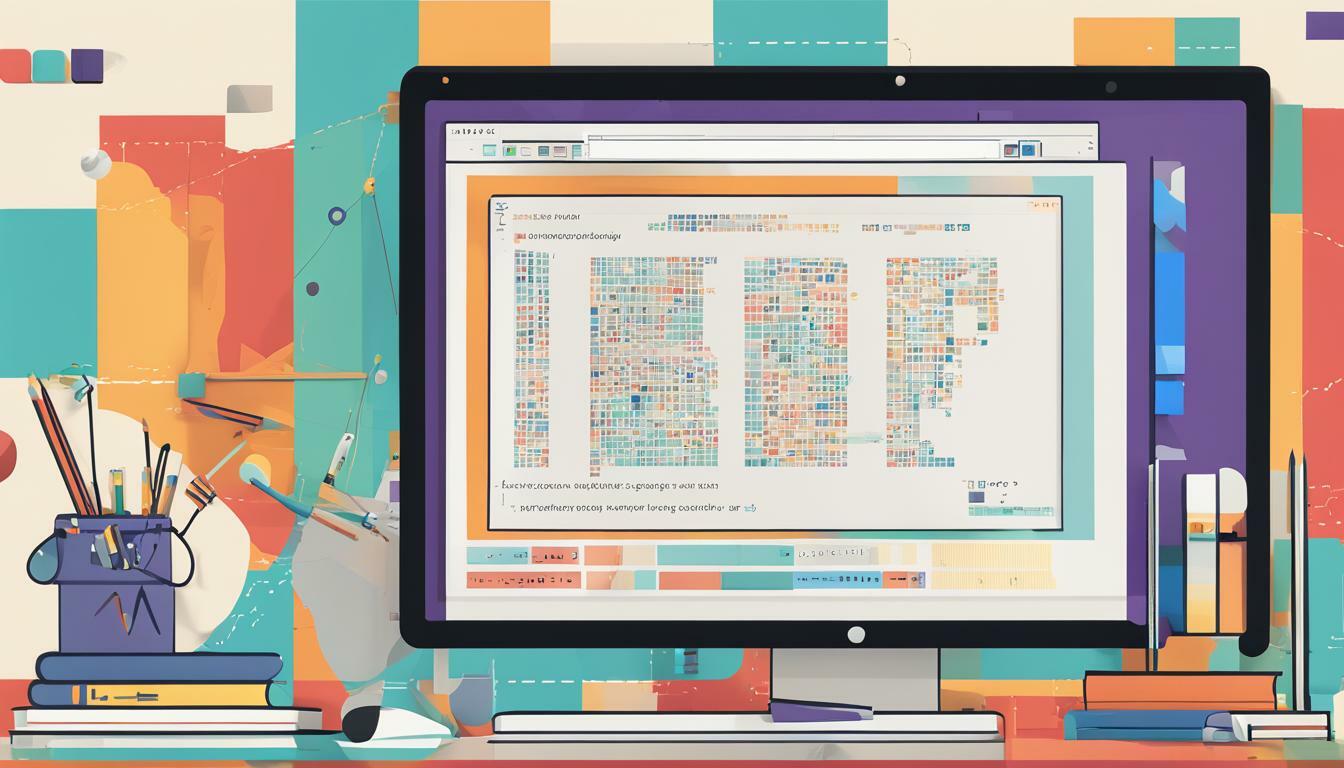
Welcome to the world of Object-Oriented Programming (OOP), where you will have the power to create modular, efficient, and reusable code. OOP is a powerful tool that allows programmers to build software using objects that interact with each other to perform specific tasks. When you master the basics of OOP, you will acquire a coding skill that will help you design complex systems and applications. In this section, we will introduce you to the fundamentals of OOP, so you can start your journey towards becoming a proficient OOP programmer.
Key Takeaways
- Object-Oriented Programming (OOP) is a powerful coding paradigm that enables you to build modular, efficient, and reusable software.
- Understanding the basics of OOP is essential to becoming a proficient OOP programmer.
- Mastering the core concepts of OOP will enhance your coding skills and unlock your potential as a programmer.
Understanding Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming paradigm that uses objects to represent and manipulate data. It differs from other programming paradigms in that it focuses on data rather than procedures or functions. OOP promotes code reusability and maintainability, making it a popular choice for software development.
At its core, OOP is based on the concept of objects. An object is a self-contained unit of data that consists of a set of attributes, or properties, and a set of methods, or functions, that operate on those properties. By encapsulating data and functionality within objects, OOP allows for more modular and organized code.
OOP has several benefits over other programming paradigms. It promotes code reuse, as objects can be reused across multiple projects or modules. It also makes code more maintainable, as changes to one object will not affect other objects. Additionally, OOP makes programs more extensible, as new objects can be easily added to the codebase without disrupting existing code.
Understanding Object-Oriented Programming
One of the key features of OOP is inheritance. Inheritance allows you to create new classes based on existing ones, with the new classes inheriting all the properties and methods of the original class. This promotes code reuse and helps organize code hierarchically.
Another essential concept in OOP is polymorphism. Polymorphism allows objects of different classes to be treated as instances of a common superclass. This enhances code flexibility and extensibility by enabling you to use objects in a more generic way.
OOP also emphasizes encapsulation, which is the practice of hiding implementation details and providing a clean interface for interacting with objects. Encapsulation makes code more modular and easier to maintain by restricting access to sensitive data and only allowing access through designated methods.
Abstraction is another fundamental concept in OOP. Abstraction allows you to focus on the essential features of an object while hiding unnecessary complexity. This enhances code modularity and maintainability by exposing only the necessary details of an object to the rest of the codebase.
In conclusion, OOP is a powerful programming paradigm that allows for more modular, maintainable, and reusable code. By understanding the key concepts of OOP, you can take your coding skills to the next level and build better software.
Key Concepts in OOP
Object-Oriented Programming (OOP) revolves around four main principles: encapsulation, inheritance, polymorphism, and abstraction. These concepts are essential building blocks for developing modular and maintainable code.
Encapsulation
Encapsulation is the process of hiding data and functionality within a class, protecting it from external interference. By accessing an object’s methods and properties, you can interact with the data it contains without directly modifying it. Encapsulation helps to keep your code organized and manageable, reducing errors and increasing reusability.
Inheritance
Inheritance is a mechanism that allows you to define a new class based on an existing class. The new class inherits the properties and methods of the parent class, which can be modified or extended to create new functionality. Inheritance enables code reuse, as it eliminates the need to rewrite code that has already been implemented in other classes.
Polymorphism
Polymorphism enables objects of different classes to be treated as instances of a common superclass. This means that you can write code that works with different objects in a generic way, without having to specify their exact class. Polymorphism enhances code flexibility and simplifies maintenance, as it allows you to add new functionality without modifying existing code.
Abstraction
Abstraction is the process of hiding unnecessary implementation details of a class and exposing only the essential features to the outside world. This simplifies the interface and makes the code more manageable and reusable. Abstraction allows you to focus on high-level design rather than low-level implementation, which promotes code modularity and maintainability.
Classes and Objects in OOP
In Object-Oriented Programming (OOP), classes and objects are the building blocks that enable you to create modular, reusable, and maintainable code. A class is a blueprint for creating objects, while an object is an instance of a class.
To define a class, you must specify its name, attributes, and methods. The attributes represent the state of the class, while the methods encapsulate the behavior of the class.
Once you have defined a class, you can create objects based on it. Objects have their own state and behavior, but they share the same structure and behavior defined by the class. You can create as many objects as you need from the same class, each with its own unique state and behavior.
Defining a Class
To define a class in OOP, you use the class keyword followed by the name of the class. Here’s an example:
<class>ObjectName = class ClassName:
# Attributes
attribute1 = value1
attribute2 = value2
…
# Methods
def method1(self):
…
def method2(self):
…
…
In the above example, the ClassName represents the name of the class, while the attribute and method names can be customized based on your requirements.
Creating Objects
To create an object in OOP, you use the <class>ObjectName = ClassName() syntax. Here’s an example:
car = Car()
In the above example, car is an object created from the Car class. You can create multiple objects using the same syntax, each with its unique state and behavior.
OOP enables you to create classes and objects that are easy to maintain and reuse. By mastering classes and objects, you can write modular and scalable code that can be extended and modified with ease.
Understanding Methods in OOP
Methods are an essential aspect of Object-Oriented Programming (OOP) and play a crucial role in encapsulating behavior within objects. A method is a block of code that defines actions to be performed on an object.
One of the primary benefits of using methods is that they enable you to organize code into reusable, modular chunks. Instead of writing the same code repeatedly, you can encapsulate it into a method and call it whenever you need it. This approach saves time and makes your code more efficient and easier to maintain.
To define a method in OOP, you must specify the method’s name, access level (public, private, or protected), return type, and any parameters it requires. Once you have defined a method, you can call it from any object of the same class.
Here’s an example of how to define a simple method in Java:
public void sayHello() {
System.out.println(“Hello, world!”);
}
In this example, we have defined a method called sayHello that takes no parameters and returns no value (void). When we call this method from an object of the same class, it will print the message “Hello, world!” to the console.
Methods can also take parameters, which enable you to pass values to a method to perform specific tasks. The method can then use the parameter values to perform calculations or manipulate data. Here’s an example of a method with parameters:
public void calculateSum(int a, int b) {
int sum = a + b;
System.out.println(“The sum of ” + a + ” and ” + b + ” is ” + sum);
}
In this example, we have defined a method called calculateSum that takes two integer parameters (a and b) and returns no value (void). The method calculates the sum of a and b and prints the result to the console.
Understanding and using methods is critical to mastering OOP. By encapsulating behavior into methods, you can write more efficient, modular, and reusable code that is easier to maintain.
Exploring Encapsulation in OOP
Encapsulation is a key concept in Object-Oriented Programming (OOP) that enables you to protect the internal workings of an object from external interference. By doing so, you can ensure that the object operates correctly, and its state remains consistent.
Encapsulation involves grouping related data and functions into a single unit, known as a class. The data is hidden from the outside world and can only be accessed through defined methods. This way, you can prevent unwanted changes to object data, and your code remains secure.
The most common way to achieve encapsulation in OOP is by defining data members as private and methods as public. Private data can only be accessed within the same class, whereas public methods can be called from outside the class to access or modify private data.
For example, suppose you have a class named “Car” that has a private data member called “mileage.” If you want to change the mileage of a car object, you would call a public method defined within the same class, such as “setMileage().” The method would perform any necessary validations and then update the mileage data member.
Encapsulation provides several benefits, such as code reusability, maintainability, and flexibility. By hiding implementation details, you can change the internal workings of a class without affecting the rest of the codebase. This way, you can make your code more modular, which translates to fewer bugs and faster development.
Moreover, encapsulation also enhances code security by preventing unauthorized access to private data. It is easier to maintain a codebase where data members are hidden from the outside world and can only be accessed through predefined methods.
Benefits of Encapsulation in OOP
The following are some of the key benefits of encapsulation in OOP:
- Protects data from unwanted modification or access
- Improves code maintenance and reusability
- Enhances code security
- Enables easy modification of class implementation without affecting other code
By leveraging encapsulation, you can write more secure, maintainable, and modular code. Encapsulation is one of the primary foundations of OOP and an essential concept for any developer to master.
Understanding Inheritance in OOP
One of the most significant benefits of Object-Oriented Programming is the ability to create new classes based on existing ones through inheritance. Inheritance allows you to reuse code and promote code organization and maintenance, making your code more efficient and easier to manage.
Inheritance works by creating a hierarchy of classes where the child classes inherit properties and methods from their parent class. The child class can then add new properties and methods or override existing ones. This enables you to create specialized versions of a class without having to rewrite the entire code.
For example, consider a program that models different types of vehicles. We can create a parent class called Vehicle, with properties such as color, weight, and speed, and methods such as start() and stop(). We can then create child classes such as Car and Motorcycle, which can inherit the properties and methods from the parent class. The child classes can also have their properties and methods unique to them, such as the number of doors for a Car or the number of wheels for a Motorcycle.
Vehicle (Parent Class) | Car (Child Class) | Motorcycle (Child Class) |
---|---|---|
Color | Color | Color |
Weight | Weight | Weight |
Speed | Speed | Speed |
Start() | Start() | Start() |
Stop() | Stop() | Stop() |
N/A | Number of Doors | Number of Wheels |
With inheritance, you can also create more complex hierarchies of classes, with multiple levels of parent and child classes. This enables you to reuse code and promote code organization and maintenance even further.
Overall, inheritance is a powerful feature of Object-Oriented Programming that enables you to create new classes based on existing ones, promoting code reuse, organization, and maintenance.
Exploring Polymorphism in OOP
Polymorphism allows objects of different classes to be treated as instances of a common superclass. This feature enhances code flexibility and extensibility, making Object-Oriented Programming a versatile coding paradigm.
Polymorphism is achieved through inheritance and method overriding. Subclasses inherit methods and attributes from their superclasses, but can also override them with their own implementations. This allows different objects to share common behaviors while providing customized functionality.
For example, consider a program that simulates different shapes. Each shape can calculate its area, but the formula for each shape is different. A superclass Shape can define a method calculateArea, which is overridden in the subclasses Circle, Square, and Rectangle, each providing its specific formula.
Shape | Circle | Square | Rectangle |
---|---|---|---|
calculateArea() | calculateArea() //uses the formula for a circle | calculateArea() //uses the formula for a square | calculateArea() //uses the formula for a rectangle |
In this way, you can treat all shapes as instances of the Shape class and call the calculateArea method, which will execute the appropriate implementation for each shape.
Polymorphism also enables the creation of generic functions that can operate on objects regardless of their specific class. This feature provides code reuse and promotes modular design.
In conclusion, polymorphism is a fundamental concept in Object-Oriented Programming that allows for flexible and extensible code. By leveraging inheritance and method overriding, polymorphism enhances code reuse and promotes modular design, making OOP a powerful coding paradigm.
Abstraction in OOP
Abstraction is a critical concept in Object-Oriented Programming (OOP) that allows you to focus on the essential features of an object while hiding unnecessary complexity. In other words, it enables you to show only what is necessary while concealing irrelevant details. This approach has significant benefits in software development, including code modularity and maintainability.
By using abstraction, you can create high-level classes that define the essential properties and behaviour of objects. These classes can serve as templates for creating more specialized subclasses, each with its own unique features. By separating these classes and their specific implementations, you can write code that is more modular and easier to maintain over time.
One of the most common ways to achieve abstraction is through abstract classes and interfaces. Abstract classes define a set of methods that must be implemented by subclasses, while interfaces serve as contracts that define specific behaviours. By using these abstractions, you can standardize the way objects interact with each other and simplify your code architecture.
Overall, abstraction is a powerful technique that can help you create more modular, maintainable, and extensible code in your OOP project. By mastering this concept, you can take your programming skills to the next level and build software that is both efficient and effective.
Conclusion
Object-Oriented Programming (OOP) is a valuable skill that every programmer should learn. By mastering the basics of OOP, you will be able to create modular, reusable, and maintainable code that can be easily extended and modified over time. Understanding OOP can also make you a more efficient and effective developer, helping you to solve complex problems with ease.
In this article, we have covered the key concepts and principles of Object-Oriented Programming. We have discussed the importance of encapsulation, inheritance, polymorphism, and abstraction in building robust and flexible code. We have also explored how to define classes and objects, and how to use methods in your code.
By applying these techniques, you can take your programming skills to the next level and create software that is both elegant and efficient. So, whether you are a beginner or an experienced programmer, now is the time to start mastering Object-Oriented Programming.
Thank you for reading, and we wish you the best of luck in your programming journey!
FAQ
Q: What is Object-Oriented Programming (OOP)?
A: Object-Oriented Programming (OOP) is a coding paradigm that organizes software design around objects, which can contain data and behavior. It focuses on the concepts of encapsulation, inheritance, polymorphism, and abstraction to build modular and reusable code.
Q: How is OOP different from other programming paradigms?
A: OOP differs from other programming paradigms by emphasizing the organization of code into objects, which allows for better code reuse, modularity, and maintenance. It also enables the creation of complex software systems by breaking them down into smaller, self-contained objects.
Q: What are the key concepts in OOP?
A: The key concepts in OOP are encapsulation, inheritance, polymorphism, and abstraction. Encapsulation is the process of hiding implementation details and providing a clean interface for interacting with objects. Inheritance allows for the creation of new classes based on existing ones, promoting code reuse and organization. Polymorphism allows objects of different classes to be treated as instances of a common superclass, enhancing code flexibility. Abstraction focuses on identifying the essential features of an object while hiding unnecessary complexity.
Q: How do classes and objects work in OOP?
A: Classes are blueprints or templates for creating objects. They define the properties (attributes) and behaviors (methods) that objects of that class can have. Objects, on the other hand, are instances of classes. They contain the actual values of the attributes and can perform actions defined by the methods.
Q: What are methods in OOP?
A: Methods are functions or procedures defined within a class that encapsulate the behavior of objects. They allow you to define actions that objects can perform. Methods can take input parameters, perform operations, and return values.
Q: How does encapsulation work in OOP?
A: Encapsulation in OOP involves hiding the internal implementation details of an object and providing a clean interface for interacting with it. It helps protect the integrity of the data by controlling access through methods and properties, ensuring that only the intended behavior is exposed to external code.
Q: What is inheritance in OOP?
A: Inheritance in OOP allows you to create new classes based on existing ones, inheriting their attributes and behaviors. This promotes code reuse and enables the creation of hierarchies where subclasses inherit characteristics from their parent class. It also simplifies code maintenance and organization.
Q: How does polymorphism work in OOP?
A: Polymorphism in OOP allows objects of different classes to be treated as instances of a common superclass. This provides flexibility in code design by allowing the same method to be used with objects of different types. It enables dynamic binding, where the appropriate method is called based on the actual object’s type at runtime.
Q: What role does abstraction play in OOP?
A: Abstraction in OOP allows you to focus on the essential features of an object while hiding unnecessary complexity. It simplifies code design by providing a high-level view of objects, their behaviors, and their interactions. Abstraction enhances code modularity, maintainability, and understanding.
Q: What are the benefits of learning OOP?
A: By mastering the basics of Object-Oriented Programming, you can build modular, reusable, and maintainable software. OOP enables better code organization, code reusability, and code extensibility. It also promotes efficient problem-solving and enhances your programming skills.